This post describes how to setup a git repository and shows the basic commands for daily work. The post will be extended over time with additional commands and tricks.
Please note that the post does not describe how to set up a remote git server. You can check for example here how that can be done: https://git-scm.com/book/en/v2/Git-on-the-Server-Setting-Up-the-Server.
Page Contents
Setting up a bare git repository
To create a blank repository you just create a new folder for your project and run "git init --bare" for the git initialisation:
git@server:~/repos $ mkdir testproject.git
git@server:~/repos $ cd testproject.git/
git@server:~/repos/testproject.git $ git init --bare
hint: Using 'master' as the name for the initial branch. This default branch name
hint: is subject to change. To configure the initial branch name to use in all
hint: of your new repositories, which will suppress this warning, call:
hint:
hint: git config --global init.defaultBranch <name>
hint:
hint: Names commonly chosen instead of 'master' are 'main', 'trunk' and
hint: 'development'. The just-created branch can be renamed via this command:
hint:
hint: git branch -m <name>
Initialized empty Git repository in /path/to/repos/testproject.git/
git@server:~/repos/testproject.git $
I did this on my central git server to additionally show some operations when working with a remote project but you can also do this just with a local project.
Cloning git repository
To clone the repository to another machine you can just run the following command.
user1@client:~/projects$ git clone gitserver:/path/to/repos/testproject.git
Clone into 'testproject' …
warning: You appear to have cloned an empty repository.
user1@client:~/projects$
Please note that gitserver is pointing to a ssh config here.
First change, commit and push
I add a simple text file to my local repository. With git add I will add it under version control and will commit with the message "First commit".
user1@client:~/projects/testproject$ echo "test" >> file.txt
user1@client:~/projects/testproject$ ls
file.txt
user1@client:~/projects/testproject$ tail file.txt
test
user1@client:~/projects/testproject$ git add .
user1@client:~/projects/testproject$ git commit -m "First commit"
[master (Root-Commit) ecbe223] First commit
1 file changed, 1 insertion(+)
create mode 100644 file.txt
user1@client:~/projects/testproject$
The change is now just committed in my local repository. To check how it works check the following drawing.
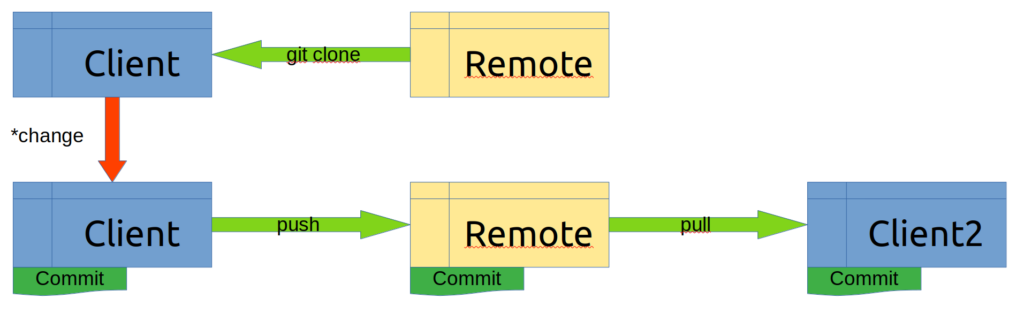
We created a new repository on the git server (Remote) and cloned it to the client. Afterwards we made a change and committed it. To make the change available to others via our git server we need to push it back.
user1@client:~/projects/testproject$ git push origin
Enumerating objects: 3, done.
Counting objects: 100% (3/3), done.
Writing objects: 100% (3/3), 225 Bytes | 225.00 KiB/s, done.
Total 3 (delta 0), reused 0 (delta 0), Pack reused 0
To gitserver:/path/to/repos/testproject.git
* [new branch] master -> master
user1@client:~/projects/testproject$
Create and work on a git branch
The branch we used so far is the master branch which usually acts as the central collection of changes in a git repository. To isolate changes (e.g. from affecting others when trying something out) you can create separate branches. To create a branch called "feature1" run
user1@client:~/projects/testproject$ git branch feature1
user1@client:~/projects/testproject$ git branch --list
feature1
* master
user1@client:~/projects/testproject$
The new branch is created but we are working still on master branch (noted by *). To switch to feature1 branch we have to checkout the branch.
user1@client~/projects/testproject$ git checkout feature1
Switched to branch 'feature1'
I create now another file called feature1.txt and commit it to the new branch.
user1@client:~/projects/testproject$ echo "feature1" >> feature1.txt
user1@client:~/projects/testproject$ git add .
user1@client:~/projects/testproject$ git commit -m "Adding Feature1"
[feature1 22f1a8a] Adding Feature1
1 file changed, 1 insertion(+)
create mode 100644 feature1.txt
user1@client:~/projects/testproject$
The feature1 branch contains now two commits:
user1@client:~/projects/testproject$ git log
commit 22f1a8ab8cbc858571429265f50e58bbfbbc736d (HEAD -> feature1)
Author: Benni <b@wdev.ch>
Date: Wed Apr 5 21:23:33 2023 +0200
Adding Feature1
commit ecbe2232341d8a612afefdaf8f7150dcf16c506b (origin/master, master)
Author: Benni <b@wdev.ch>
Date: Wed Apr 5 21:00:14 2023 +0200
First commit
user1@client:~/projects/testproject$
The master branch is not affected by this change, see here.
user1@client:~/projects/testproject$ git checkout master
Switched to branch 'master'
Your branch is up to date with 'origin/master'.
user1@client:~/projects/testproject$ git log
commit ecbe2232341d8a612afefdaf8f7150dcf16c506b (HEAD -> master, origin/master)
Author: Benni <b@wdev.ch>
Date: Wed Apr 5 21:00:14 2023 +0200
First commit
user1@client:~/projects/testproject$
Leave a Reply